In HTML, Javascript is often used in conjunction with HTML and CSS to create interactive features, perform calculations, manipulate the content of a web page, and handle user interactions.
HTML Javascript
The HTML <script> tag is used to embed or reference JavaScript code within an HTML document.
It allows you to add interactive and dynamic functionality to your web pages. The <script> tag can be placed in the <head> or <body> section of an HTML document.
Here are a few important aspects of the <script> tag:
Inline JavaScript:
You can include JavaScript code directly within the <script> tags. For example:
<script>
// JavaScript code goes here
</script>
External JavaScript file
Instead of inline code, you can also reference an external JavaScript file using the src attribute of the <script> tag. For example:
<script src="script.js"> </script>
In this case, the JavaScript code is stored in a separate file called "script.js", and it will be executed when the browser encounters this <script> tag.
Placement
The <script> tag can be placed in the <head> section or at the end of the <body> section. Placing it in the <head> section allows the script to be loaded and executed before the entire page is rendered. Placing it at the end of the <body> section allows the page content to be loaded first, improving the page's perceived loading speed.
Document Ready
To ensure that the JavaScript code executes after the HTML document is fully loaded, you can wrap your code inside an event listener for the "DOMContentLoaded" event. For example:
<script>
document.addEventListener('DOMContentLoaded', function() {
// JavaScript code that runs after the HTML document is loaded
});
</script>
This ensures that the JavaScript code inside the event listener is executed only when the HTML document is ready.
Attributes
The <script> tag also supports additional attributes like async and defer for controlling the script's loading and execution behavior.
The async attribute allows the script to be loaded asynchronously without blocking the HTML parsing, while the defer attribute defers the script execution until the HTML document is fully parsed.
It's important to note that improper use of JavaScript code can affect the performance and behavior of your web page. Always ensure that your code is properly written, tested, and adheres to best practices.
Now, let's dive into five examples that demonstrate the combination of HTML and JavaScript:
Button Click Counter
<!DOCTYPE html>
<html>
<head>
<script>
function incrementCounter() {
var counterElement = document.getElementById("counter");
var currentCount = parseInt(counterElement.innerHTML);
counterElement.innerHTML = currentCount + 1;
}
</script>
</head>
<body>
<p>Button Click Counter</p>
<p>Count: <span id="counter">0</span></p>
<button onclick="incrementCounter()">Increment</button>
</body>
</html>
Button Click Counter
Count: 0
In this example, a JavaScript function incrementCounter() is defined. When the button is clicked, this function is called, and the count displayed on the web page increases by one.
Form Validation
<!DOCTYPE html>
<html>
<head>
<script>
function validateForm() {
var name = document.getElementById("name").value;
if (name === "") {
alert("Please enter your name.");
return false;
}
return true;
}
</script>
</head>
<body>
<p>Form Validation Example</p>
<form onsubmit="return validateForm()">
<label for="name">Name:</label>
<input type="text" id="name" required>
<input type="submit" value="Submit">
</form>
</body>
</html>
Form Validation Example
In this example, the JavaScript function validateForm() is called when the form is submitted. It checks if the name input field is empty and displays an alert if it is.
Dynamic Content Update
<!DOCTYPE html>
<html>
<head>
<script>
function updateContent() {
var contentElement = document.getElementById("content");
contentElement.innerHTML = "New content!";
}
</script>
</head>
<body>
<p>Dynamic Content Example</p>
<p id="content">Initial content</p>
<button onclick="updateContent()">Update Content</button>
</body>
</html>
Dynamic Content Example
Initial content
In this example, the JavaScript function updateContent() changes the content of the <p> element with the id "content" to "New content!" when the button is clicked.
Mouse Events
<!DOCTYPE html>
<html>
<head>
<script>
function handleMouseOver() {
var element = document.getElementById("target");
element.style.backgroundColor = "red";
}
function handleMouseOut() {
var element = document.getElementById("target");
element.style.backgroundColor = "";
}
</script>
</head>
<body>
<p>Mouse Events Example</p>
<div id="target" onmouseover="handleMouseOver()" onmouseout="handleMouseOut()">
Hover over me!
</div>
</body>
</html>
Mouse Events Example
In this example, the JavaScript functions handleMouseOver() and handleMouseOut() are triggered when the mouse hovers over and leaves the <div> element respectively. These functions change the background color of the element.
Dynamic Image Change
<!DOCTYPE html>
<html>
<head>
<script>
function changeImage() {
var imageElement = document.getElementById("image");
imageElement.src = "new-image.jpg";
}
</script>
</head>
<body>
<p>Mouse Events Example</p>
<div id="target" onmouseover="handleMouseOver()" onmouseout="handleMouseOut()">
Hover over me!
</div>
</body>
</html>
Dynamic Image Change Example
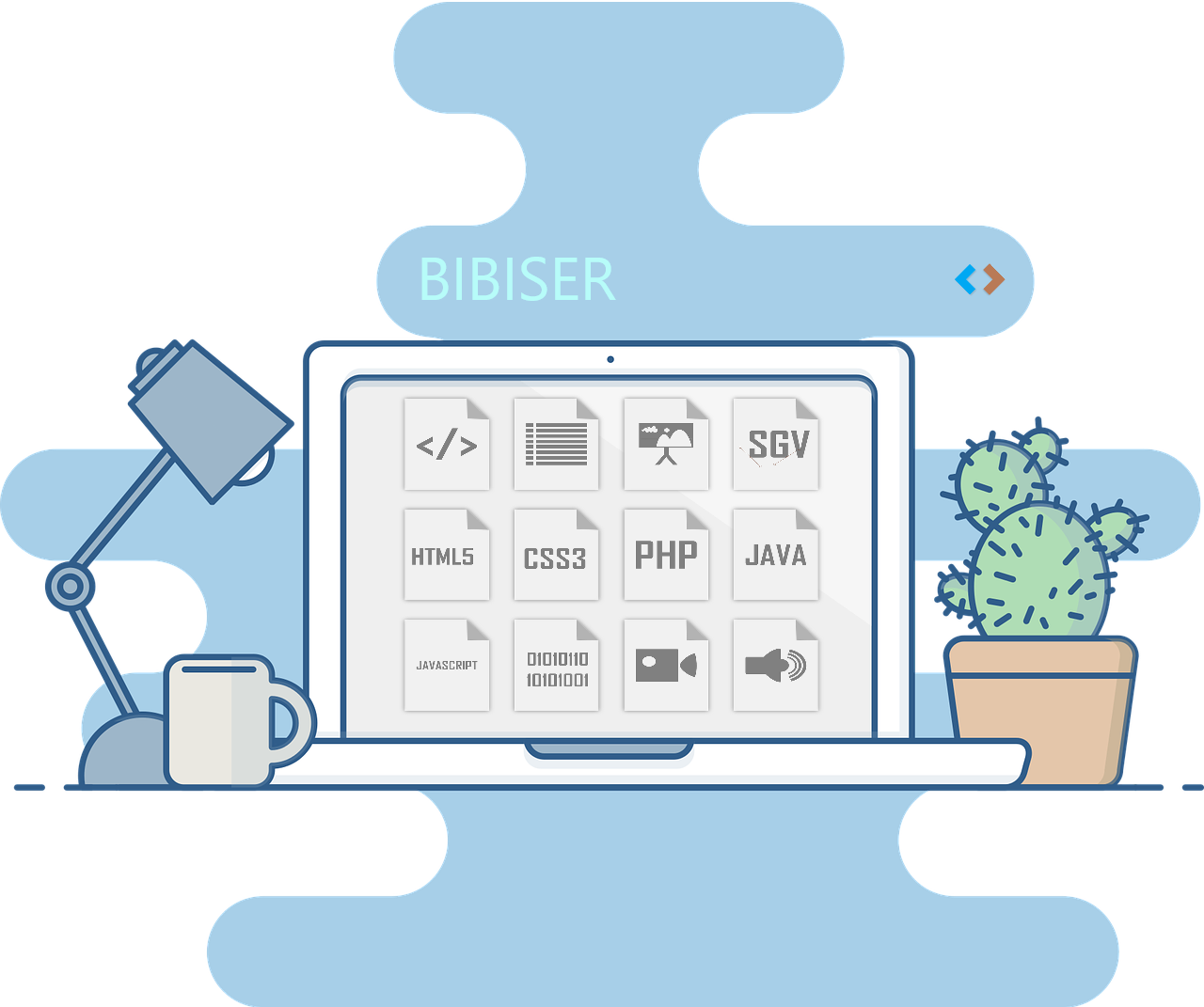
In this example, the JavaScript function changeImage() is called when the button is clicked. It updates the source (src) attribute of the <img> element, dynamically replacing the displayed image with a new one.
These examples demonstrate the combination of HTML and JavaScript to add interactivity, perform actions based on user input, update content dynamically, and handle events. Feel free to modify and experiment with these examples to understand how HTML and JavaScript work together to create dynamic web pages.