DYNAMICALLY UPDATING a web page refers to the process of modifying its content, structure, or appearance in real-time using JavaScript.
Javascript dynamic web page updates
This allows for interactive and responsive user experiences by manipulating HTML elements, updating text and images, adjusting styles, and responding to user actions.
JavaScript provides various techniques such as DOM manipulation, Event handling, AJAX requests, and Data binding to achieve dynamic updates.
By leveraging these capabilities, developers can create dynamic web pages that adapt and change dynamically based on user input or external data sources, enhancing interactivity and user engagement.
Updating Text Content
<button onclick="changeText()">Change Text</button>
<p id="myText">Original Text</p>
<script>
function changeText() {
document.getElementById("myText").textContent = "Updated Text";
}
</script>
In this example, we have a button and a paragraph (<p>) element with the ID myText. When the button is clicked, the changeText() function is called. The function uses the textContent property to update the text content of the paragraph to "Updated Text".
Original Text
Modifying Styles
<button onclick="changeColor()">Change Color</button>
<p id="ExampleText">Text with Style</p>
<script>
function changeColor() {
var element = document.getElementById("ExampleText");
element.style.color = "red";
element.style.fontWeight = "bold";
}
</script>
In this example, when the button is clicked, the changeColor() function is executed. The function retrieves the element with the ID myText and modifies its style properties. It changes the text color to red and makes the text bold by updating the color and fontWeight CSS properties, respectively.
Text with Style
Handling User Input
<input type="text" id="myInput" oninput="updateText()">
<p id="displayText">Type something...</p>
<script>
function updateText() {
var input = document.getElementById("myInput");
var display = document.getElementById("displayText");
display.textContent = input.value;
}
</script>
In this example, we have an input field (<input>) with the ID myInput and a paragraph with the ID displayText. The oninput attribute is used to call the updateText() function whenever the user types into the input field. The function retrieves the input value and updates the text content of the paragraph to reflect the input.
Type something...
These examples demonstrate different ways to dynamically update web pages using JavaScript and HTML. By manipulating text content, modifying styles, and responding to user input, you can create dynamic and interactive web pages that provide a more engaging user experience.
Showing and Hiding Elements
<button onclick="toggleElement()">Toggle Element</button>
<div id="myElement" style="display: none;">
<p>This element can be toggled.</p>
</div>
<script>
function toggleElement() {
var element = document.getElementById("myElement");
if (element.style.display === "none") {
element.style.display = "block";
} else {
element.style.display = "none";
}
}
</script>
In this example, we have a button that toggles the visibility of a <div> element with the ID myElement. Initially, the element is hidden (display: none;). When the button is clicked, the toggleElement() function is called. It checks the current display style of the element and toggles it between "none" and "block" to show or hide the element accordingly.
Adding and Removing Elements
<button onclick="addListItem()">Add List Item</button>
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
</ul>
<script>
function addListItem() {
var list = document.getElementById("myList");
var newItem = document.createElement("li");
newItem.textContent = "New Item";
list.appendChild(newItem);
}
</script>
In this example, we have a button that adds a new list item to an unordered list (<ul>) with the ID myList. When the button is clicked, the addListItem() function is called. It creates a new <li> element, sets its text content to "New Item", and appends it to the list.
- Item 1
- Item 2
Dynamic Image Source
<button onclick="changeImage()">Change Image</button>
<img id="myImage" src="image1.jpg" alt="Image">
<script>
function changeImage() {
var image = document.getElementById("myImage");
image.src = "image2.jpg";
}
</script>
In this example, we have an image (<img>) element with the ID myImage and an initial source set to "image1.jpg". When the button is clicked, the changeImage() function is called, which modifies the src attribute of the image element to "image2.jpg". This updates the image displayed on the page.
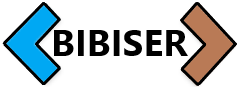
Resources for Further Learning
Explore the possibilities and create unique effects on your web pages by using the interactive code editor
HTML
Write and customize HTML code
CSS
Add style and design to your web pages with CSS
JavaScript
Enhance interactivity with JavaScript code